Creating PDFs in .NET: Best Methods and Libraries
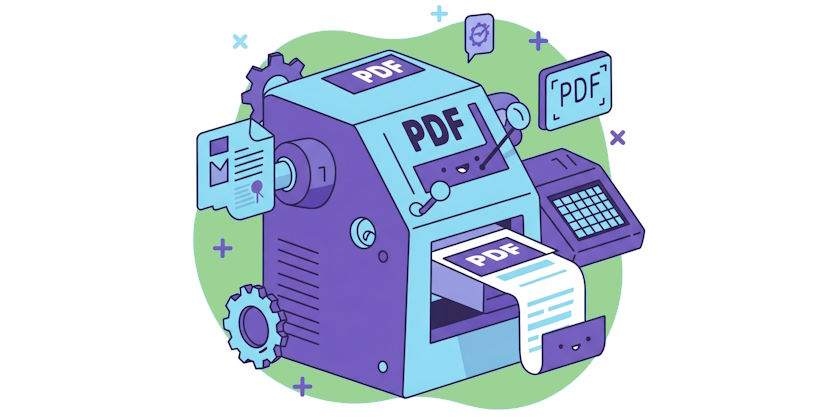
Introduction
Portable Document Format (PDF) is one of the most widely used file formats on the web. It is commonly used for creating printable, read-only documents such as reports, invoices, contracts, and manuals. As a .NET developer, I have worked on many web applications that required generating PDF files.
If you need to create a PDF file from your .NET code, there are a few different ways to do it. You can use a nuget package to create a PDF directly in code or you can create a HTML document and then convert it to a PDF document.
Each method has its own advantages and disadvantages, so it’s important to choose the one that best fits your project’s needs.
Lets look at some of the options for creating PDF files with Microsoft .NET.
Questions to Consider Before Choosing a Method
Before selecting a PDF generation approach, consider the following factors:
- How many PDFs do you need to generate per hour? Some methods are slower than others.
- Is memory usage a concern? Some approaches consume more memory than others.
- Are you willing to pay for a commercial license? Some libraries require a paid license for advanced features.
- Is deployment size a factor? Some solutions require large dependencies that can affect your application’s performance and hosting requirements.
.NET PDF Libraries
For more control over PDF generation, you can use .NET libraries that allow you to create and manipulate PDF files directly in your code. This method is highly efficient, as it avoids the overhead of rendering HTML and can generate PDFs entirely in memory.
Advantages:
- Full control over the structure and content of the PDF.
- Can be memory-efficient since it does not rely on external executables.
- Easy to deploy within a .NET application.
Disadvantages:
- Requires learning the library’s API, which can have a steep learning curve.
- Some libraries have licensing restrictions, with free usage limits and paid plans for higher usage.
Popular .NET PDF Libraries:
- Open-source library for creating and modifying PDF files.
- Supports Encryption, Digital signatures and Archiving (PDF/A)
- Modern, fluent API for creating PDFs programmatically.
- Free for small-scale use but requires a license for commercial projects.
- Powerful library for PDF generation and manipulation.
- Free for basic use, but requires a commercial license for advanced features.
And there are many other commerical products.
HTML to PDF Conversion
One of the easiest ways to create a PDF is to generate the content as an HTML document and then convert it to a PDF file. This method is useful because it allows you to use familiar web technologies like HTML and CSS to format your document.
Advantages:
- You can use your existing knowledge of HTML and CSS for styling.
- The PDF can match the look and feel of your web application by using the same styles and themes.
- It is relatively easy to implement.
Disadvantages:
- Not all CSS properties are supported in the conversion process.
- The rendered PDF may not look exactly the same as the original HTML document.
- Handling headers, footers, and page breaks can be difficult if precise formatting is required.
- Can require an external program to do the conversion, increasing deployment complexity.
Popular HTML to PDF Libraries:
Scryber
An complete, pdf creation library that can work by converting XHTML documents to PDF or by building the document structure in code.
PeachPDF
A HTML to PDF conversion library that does not have any external dependencies. Review its features as it might not support complex CSS and layout.
Wkhtmltopdf
wkhtmltopdf was a popular option but the project has been archived for a few years and should not be used for new projects. It is based on the WebKit browser engine.
- Works well for simple HTML without complex styles.
- Requires an external executable to be installed on the server.
- Some .NET wrapper libraries, like DinkToPdf, make integration easier.
Headless Browser Approach
Another approach is to use a headless browser, such as Chromium, to render a webpage and export it as a PDF. This method provides better rendering accuracy compared to Wkhtmltopdf.
Advantages:
- Uses the full power of modern browsers to render HTML and CSS accurately.
- Provides better control over styling and layout.
- Can handle JavaScript-based content.
Disadvantages:
- Consumes more memory and CPU resources, making it less efficient for large-scale PDF generation.
- Requires a large deployment size since it includes a full browser engine.
- Can sometimes have stability issues.
Popular Headless Browser Solutions:
- These libraries allow automation of the Chromium browser.
- Can be used to generate PDFs by rendering an HTML page and exporting it.
- Need to deploy a version of the browser with your application which makes deployments larger and take longer.
- A containerized version of Chromium with an API for document conversion.
- Useful for microservices-based architectures.
- More infrastructure to maintain.
Other options
There are also other options to investigate like
- PDF Generation APIs, where you send HTML to a hosted service and it returns a PDF document.
- Client-side JavaScript PDF generation e.g. jsPDF
Conclusion
Choosing the right method for generating PDFs in .NET depends on your project’s requirements. If you need a simple solution and are comfortable with HTML, using an HTML-to-PDF conversion tool may be the best choice. If you need precise control over the document’s content and structure, consider headless browser solutions. For large-scale applications with high-performance needs, using a .NET PDF library would be a better option.
Each approach has trade-offs, so carefully evaluate your needs before deciding which method to use. Hopefully, this guide helps you make an informed choice when working with PDFs in .NET!